SFS2X Docs / ExamplesAndroid / java-connector
» Java Connector
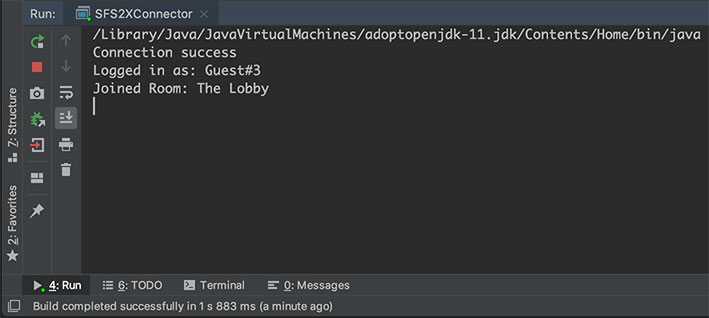
» Overview
The following example provides a simple template with which you can create more complex Java-based SFS2X clients. The Connector example shows the basics of establishing a connection, logging in the server, sending requests and handling the respective responses.
In order to be able to talk to the server, the client must successfully execute two steps:
- Establish a TCP connection
- Log into an existing Zone
A connection allows client and server to exchange messages. Once this is created we can log in the server, specifying which Zone we want to join. A Zone simply represent an application name. Since SmartFoxServer can run multiple applications at the same time, we need to specify which one we want to interact with.
By default every newly installed SmartFoxServer comes with a default Zone called BasicExamples which can be used to test our API examples.
>> DOWNLOAD the source files <<
» Installation
» Source code setup
The example sources and assets are contained in a project file and don't require a specific setup. However you will need IntelliJ IDEA (which is freely downloadable) to open the project. From IDEA's File menu choose Open and navigate to the project folder, then click Open.
» Running the example
In order to run the application follow these steps:
- Make sure SmartFoxServer 2X is running
- Open the client project in IDEA
-
From the main menu choose Run > Run 'SFS2XConnector' or hit the small Run button in the top icon bar.
» The Java code
public class SFS2XConnector { SmartFox sfs; ConfigData cfg; public SFS2XConnector() { // Configure client connection settings cfg = new ConfigData(); cfg.setHost("localhost"); cfg.setPort(9933); cfg.setZone("BasicExamples"); cfg.setDebug(false); // Set up event handlers sfs = new SmartFox(); sfs.addEventListener(SFSEvent.CONNECTION, this::onConnection); sfs.addEventListener(SFSEvent.CONNECTION_LOST, this::onConnectionLost); sfs.addEventListener(SFSEvent.LOGIN, this::onLogin); sfs.addEventListener(SFSEvent.LOGIN_ERROR, this::onLoginError); sfs.addEventListener(SFSEvent.ROOM_JOIN, this::onRoomJoin); // Connect to server sfs.connect(cfg); } ... public static void main(String[] args) { new SFS2XConnector(); } }
Our main() method creates an instance of the SFS2XConnector class, which in turn sets up the parameters for our connection using the ConfigData object.
Here we provide the address of the server we want to connect to (could be either a domain name or an IP address) and the TCP port number, which by default is 9933. If you don't plan to change this value you can also omit it in the ConfigData object, for brevity.
Also, we can specify the Zone we want to use at login time, and the debug flag which outputs the whole packet exchange between client and server in the standard output, if needed. You can experiment with this if you're interested.
» Handling events
Next we create the main client API object, the SmartFox class, and we add a number of event listeners that will trigger when the server replies to specific requests. For example the SFSEvent.CONNECTION event is fired in response to a connection attempt, and we can delegate a different method to each event we're interested to listening to.
Here we can see in detail how event handlers work:
private void onConnection(BaseEvent evt) { boolean success = (boolean) evt.getArguments().get("success"); if (success) { System.out.println("Connection success"); sfs.send(new LoginRequest("")); } else System.out.println("Connection Failed. Is the server running?"); } private void onConnectionLost(BaseEvent evt) { System.out.println("-- Connection lost --"); } private void onLogin(BaseEvent evt) { System.out.println("Logged in as: " + sfs.getMySelf().getName()); sfs.send(new JoinRoomRequest("The Lobby")); } private void onLoginError(BaseEvent evt) { String message = (String) evt.getArguments().get("errorMessage"); System.out.println("Login failed. Cause: " + message); } private void onRoomJoin(BaseEvent evt) { Room room = (Room) evt.getArguments().get("room"); System.out.println("Joined Room: " + room.getName()); }
Each method receives an event object (of type BaseEvent) which carries a number of parameters specific for that event. For example we can see that the onConnection event handler extracts a parameter called success to verify if the connection attempt worked.
In order to learn which parameters each event provides, you can consult the documentation for the Java Client API, by checking the SFSEvent class.
Back to our onConnection event handler, we can check if the connection was successful and proceed by sending a login request.
sfs.send(new LoginRequest(""));
The LoginRequest object can take several parameters:
- name: the account user name
- password: the account password
- zone: the Zone name to use
In our example we are just sending an empty user name, with no password or Zone name. The reason for this is that by default there is no server side check for user credentials. This can be implemented separately.
We can choose to either provide a unique name or send an empty string and let the server assign us a username, which will use the format Guest#NN where NN is a auto-increment value.
Also we don't provide a Zone name, because we already specified it via the ConfigData object.
» Testing the example
If you are running the example and SmartFoxServer on the same machine you can run the Example to see it in action and experiment with it.
If client and server are running on different machines you will need to change this line:
cfg.setHost("localhost");
Instead of localhost (which is the domain name of your local machine) you will need to replace it with the domain name or IP address of the machine running the server.
Example: if the server is running on another computer in your local network you need to know the IP address of that machine in the network. This can be done by launching a terminal window (Linux/macOS) or command prompt (Windows) and typing:
- (Linux/macOS): ifconfig
- (Windows 7/8/10): ipconfig
Then replace the localhost value in ConfigData with the IP address you have found.
» Creating a new SFS2X Client project
Now that you have learned the basics of connecting and interacting with SmartFoxServer you can experiment with the API by creating your own projects.
In order to do so you will need a couple of things:
- Your favorite Java IDE
- A local SmartFoxServer running
- The Java/Android client API
Once you have created a new Java project in your IDE, you can unzip the client API and add all its jar files as build dependencies. If you are not sure how to proceed with this last step make sure to consult your IDE's documentation. Typically every IDE has a Project Settings section where you can specify your build dependencies.